1.Vehicle Purchase
Instructions
In this exercise, you are going to write some code to help you prepare to buy a vehicle.
You have three tasks, one to determine if you need a license, one to help you choose between two vehicles and one to estimate the acceptable price for a used vehicle.
1. Determine if you will need a driver’s license
Some vehicle kinds require a driver’s license to operate them. Assume only the kinds "car"
and "truck"
require a license, everything else can be operated without a license.
Implement the NeedsLicense(kind)
function that takes the kind of vehicle and returns a boolean indicating whether you need a license for that kind of vehicle.
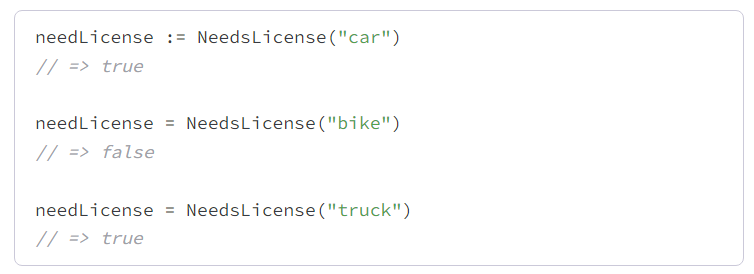
2. Choose between two potential vehicles to buy (dictionary order)
You evaluate your options of available vehicles. You manage to narrow it down to two options but you need help making the final decision. For that, implement the function ChooseVehicle(option1, option2)
that takes two vehicles as arguments and returns a decision that includes the option that comes first in lexicographical order.
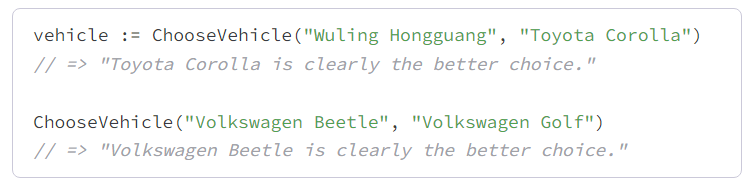
3. Calculate an estimation for the price of a used vehicle
Now that you made a decision, you want to make sure you get a fair price at the dealership. Since you are interested in buying a used vehicle, the price depends on how old the vehicle is. For a rough estimate, assume if the vehicle is less than 3 years old, it costs 80% of the original price it had when it was brand new. If it is at least 10 years old, it costs 50%. If the vehicle is at least 3 years old but less than 10 years, it costs 70% of the original price.
Implement the CalculateResellPrice(originalPrice, age)
function that applies this logic using if
, else if
and else
(there are other ways if you want to practice). It takes the original price and the age of the vehicle as arguments and returns the estimated price in the dealership.
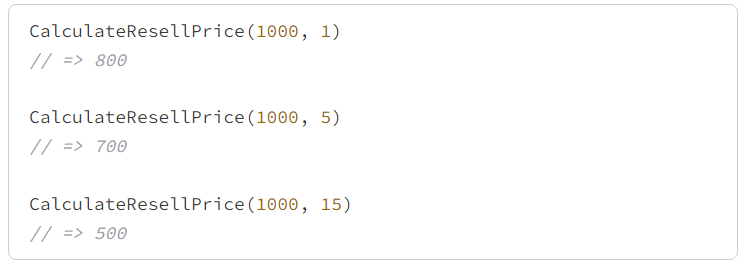
Note the return value is a float64
.
2. Bird Watcher
Instructions
You are an avid bird watcher that keeps track of how many birds have visited your garden. Usually you use a tally in a notebook to count the birds, but to better work with your data, you’ve digitalized the bird counts for the past weeks.
1. Determine the total number of birds that you counted so far
Let us start analyzing the data by getting a high level view. Find out how many birds you counted in total since you started your logs.
Implement a function TotalBirdCount
that accepts a slice of int
s that contains the bird count per day. It should return the total number of birds that you counted.

2. Calculate the number of visiting birds in a specific week
Now that you got a general feel for your bird count numbers, you want to make a more fine-grained analysis.
Implement a function BirdsInWeek
that accepts a slice of bird counts per day and a week number.
It returns the total number of birds that you counted in that specific week. You can assume weeks are always tracked completely.

3. Fix a counting mistake
You realized that all the time you were trying to keep track of the birds, there was one bird that was hiding in a far corner of the garden.
You figured out that this bird always spent every second day in your garden.
You do not know exactly where it was in between those days but definitely not in your garden.
Your bird watcher intuition also tells you that the bird was in your garden on the first day that you tracked in your list.
Given this new information, write a function FixBirdCountLog
that takes a slice of birds counted per day as an argument and returns the slice after correcting the counting mistake.
