Gopher’s Gorgeous Lasagna
Instructions
In this exercise you’re going to write some code to help you cook a brilliant lasagna from your favorite cooking book.
You have four tasks, all related to the time spent cooking the lasagna.
1. Define the expected oven time in minutes
Define the OvenTime
constant with how many minutes the lasagna should be in the oven. According to the cooking book, the expected oven time in minutes is 40:

2. Calculate the remaining oven time in minutes
Define the RemainingOvenTime()
function that takes the actual minutes the lasagna has been in the oven as a parameter and returns how many minutes the lasagna still has to remain in the oven, based on the expected oven time in minutes from the previous task.
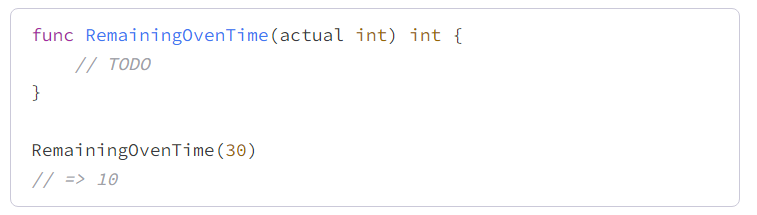
3. Calculate the preparation time in minutes
Define the PreparationTime
function that takes the number of layers you added to the lasagna as a parameter and returns how many minutes you spent preparing the lasagna, assuming each layer takes you 2 minutes to prepare.
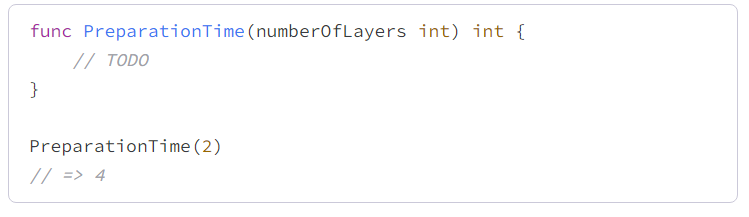
4. Calculate the elapsed working time in minutes
Define the ElapsedTime
function that takes two parameters: the first parameter is the number of layers you added to the lasagna, and the second parameter is the number of minutes the lasagna has been in the oven. The function should return how many minutes in total you’ve worked on cooking the lasagna, which is the sum of the preparation time in minutes, and the time in minutes the lasagna has spent in the oven at the moment.
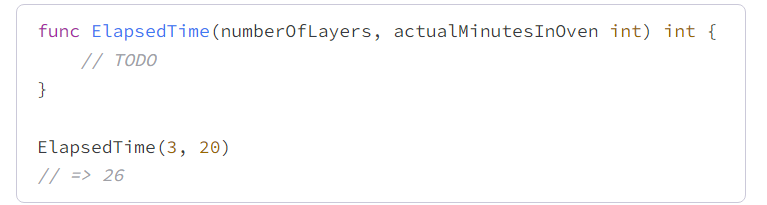
Gopher’s Cars Assemble (Challenge)
Instructions
In this exercise you’ll be writing code to analyze the production in a car factory
1. Calculate the number of working cars produced per hour
The cars are produced on an assembly line. The assembly line has a certain speed, that can be changed. The faster the assembly line speed is, the more cars are produced. However, changing the speed of the assembly line also changes the number of cars that are produced successfully, that is cars without any errors in their production.
Implement a function that takes in the number of cars produced per hour and the success rate and calculates the number of successful cars made per hour. The success rate is given as a percentage, from 0
to 100
:

Note: the return value should be a float64
.
2. Calculate the number of working cars produced per minute
Implement a function that takes in the number of cars produced per hour and the success rate and calculates how many cars are successfully produced each minute:

Note: the return value should be an int
.
3. Calculate the cost of production
Each car normally costs $10,000 to produce individually, regardless of whether it is successful or not. But with a bit of planning, 10 cars can be produced together for $95,000.
For example, 37 cars can be produced in the following way: 37 = 3 x groups of ten + 7 individual cars
So the cost for 37 cars is: 3*95,000+7*10,000=355,000
Implement the function CalculateCost
that calculates the cost of producing a number of cars, regardless of whether they are successful:
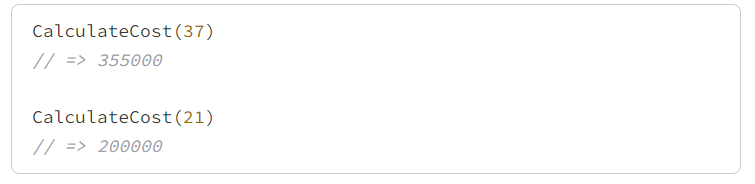
Note: the return value should be an uint
.